In one of my application i have to create it using swift technology , so i here describe you the basics of swift as well the problems that i faced during making that app
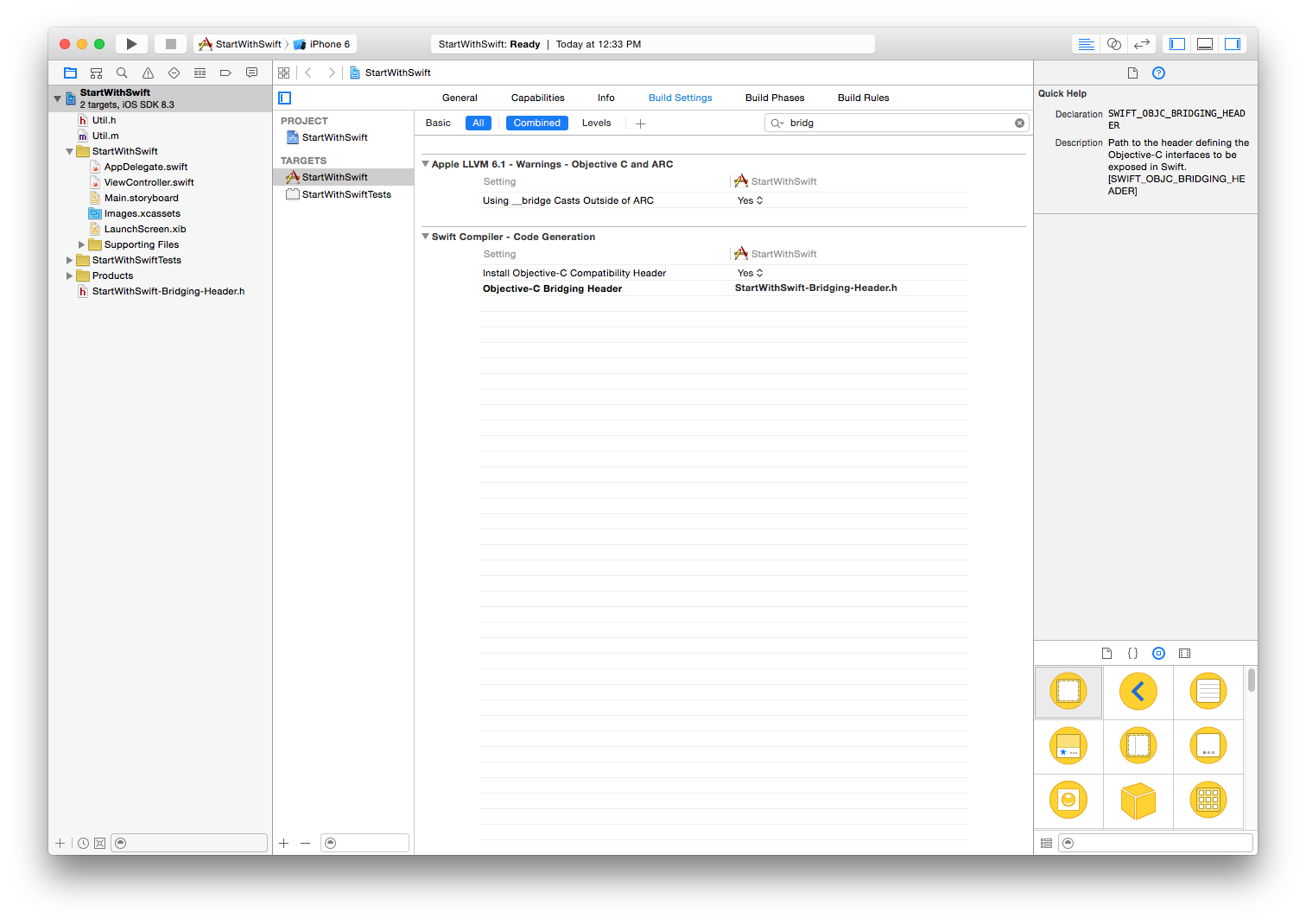
Some of the basic rules when convert objective c code to swift
What is it!!!!!!!!!!!
One of the Hazard!!!! How to use existing objective C classes in swift project
I can give very nice explanation how to use existing objective c classes in your project :)
1> Whenever you are adding one existing class in your project Xcode
will ask…like this way If you give YES then you can see one bridging header will added in your project
2> Now if you have not added bridging file then you can add this way very easily.
add a new .h file to your project and name it <#YourProjectName#>-Bridging-Header.h
you have to give Objective c bridge header in build settings like this way :
you can give path like this
$(SRCROOT)/Folder/Folder/<#YourProjectName#>-Bridging-Header.h
In StartWithSwift-Bridging-Header you can define your objective c file like this way #import "MBProgressHUD.h"
Now you can use in swift file MBProgressHUD code like this way:
class SignInViewCntrl: UIViewController,MBProgressHUDDelegate,Rest_APIDelegate{
@IBOutlet var focusField : UITextField!
you can use MBProgressHUD open source code Progress hood this way:
MBProgressHUD *hud = [[self alloc] initWithView:view];
hud.removeFromSuperViewOnHide = YES;
vice versa you can even use your own swift file in objective C File:
Some of the basic rules when convert objective c code to swift
1) Notice that when creating a string literal to assign to the string variable, we don’t need the “@” for Swift.
In Objective C We are not using @ for strings
In Swift We are using let for creating constants
2) We are using let to create a constant
Use let to make a constant and var to make a variable.
let myConstant = 42
Values are never implicitly converted to another type. If you need to convert a value to a different type, explicitly make an instance of the desired type.
Example of this :
let label = "The width is "
let width = 94
let widthLabel = label + String(width)
Use \() to include a floating-point calculation in a string and to include someone’s name in a greeting.
3) you can create the arrays using the []
var shoppingList = ["catfish", "water", "tulips", "blue paint"]
shoppingList[1] = "bottle of wate”
var occupations = [ "Malcolm": "Captain",
"Kaylee": "Mechanic", ]
occupations["Jayne"] = "Public Relations"
4) difference in datatype
in swift you have to use Bool not BOOL
5) Make a class
Objective C
@implementation Shape
{
int numberOfSides;
}
-(NSString*) simpleDescription {
return [NSString stringWithFormat:@"A shape with (%d) sides.",numberOfSides];
}
Swift
class Shape {
var numberOfSides = 0
func simpleDescription() -> String {
return "A shape with \(numberOfSides) sides."
}
}
Create an instance of a class by putting parentheses after the class name. Use dot syntax to access the properties and methods of the instance.
var shape = Shape()
shape.numberOfSides = 7
var shapeDescription = shape.simpleDescription()
6) Calling a method:
Objective c
SpecificCar *myCar = [[SpecificCar alloc] init];
[myCar getOdometerReading];
|
Swift
var myCar = SpecificCar()
myCar.getOdometerReading()
7) Id is replaced with AnyObject
In Swift Id is replaced with AnyObject
8)Enums in Swift
enum MESSAGE_DURATION : Int {
case MSG_DURATION_SHORT=2
case MSG_DURATION_LONG=4
}
Here we are using case in swift we are not applying , after case MSG_DURATION_SHORT=2
To access enum :
MESSAGE_DURATION.MSG_DURATION_SHORT
9) You can print the current value of a constant or variable with the println function:
println(friendlyWelcome)
10) Collection Types in swift
Swift provides three primary collection types, known as arrays, sets, and dictionaries,
Arrays, sets, and dictionaries in Swift are always clear about the types of values and keys that they can store. This means that you cannot insert a value of the wrong type into a collection by mistake.
Arrays
Creating a empty array using this way:
var someInts = [Int]()
someInts.append(3) //it add one object to array
someInts += [4]
you can declare array like this way
1>
var threeDoubles = [Double](count: 3, repeatedValue: 0.0)
Here the default value is 0.0
2>
var shoppingList: [String] = ["Eggs", "Milk"]
you can check array is empty or not this way:
if shoppingList.isEmpty(){
}
Set
A set stores distinct values of the same type in a collection with no defined ordering.
you can perform the same operation as in array for more info you can check
Its very broad concept we can include it in next tutorial.
11) Optional Chaining
Optional Chaining allows us to chain multiple optionals together using dot-syntax, and conditionally check if each value in the chain exists. The optional chaining operator is:?.
By virtue of being optional It simple means In the code below,
john
has a residence
property value of nil
:class Person {
var residence: Residence?
}
// Constant string that is declared as an optional.
let constantOptional: String? = "Test"
// Variable string that is declared as an optional.
var variableOptional: String? = "Test"A runtime error is thrown if a non-optional constant/variable ever becomes nilWith optionals, testing is slightly simplified, as we don’t have to explicitly add a character to test for the non-existence of our testVar variable (see example block):var testVar: String? = myRandomFunction() // May return nil
if testVar { // testVar is not nil
println("testVar is equal to \(testVar!)")
} else {
println("testVar is equal to nil")
}